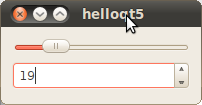
create a new class, InterClass.
interclass.h
#ifndef INTERCLASS_H
#define INTERCLASS_H
#include <QtGui>
#include <QObject>
class InterClass : public QObject
{
Q_OBJECT
public:
explicit InterClass(QObject *parent, QSlider *slider, QSpinBox *spinBox);
signals:
void spinBoxValueChanged(int value);
void sliderValueChanged(int value);
public slots:
void setValue(int value);
private:
QSlider *parentSlider;
QSpinBox *parentSpinBox;
};
#endif // INTERCLASS_H
interclass.cpp
#include <QtGui>
#include "interclass.h"
InterClass::InterClass(QObject *parent, QSlider *slider, QSpinBox *spinBox) :
QObject(parent)
{
parentSlider = slider;
parentSpinBox = spinBox;
QObject::connect(this, SIGNAL(sliderValueChanged(int)), parentSpinBox, SLOT(setValue(int)));
QObject::connect(this, SIGNAL(spinBoxValueChanged(int)), parentSlider, SLOT(setValue(int)));
}
void InterClass::setValue(int value)
{
if((QObject::sender()) == parentSlider){
emit sliderValueChanged(value);
}
else{
emit spinBoxValueChanged(value);
}
}
main.cpp
#include <QtGui>
#include "interclass.h"
int main(int argc, char* argv[])
{
QApplication app(argc, argv);
QWidget* mainwindow = new QWidget();
QVBoxLayout* mainlayout = new QVBoxLayout();
QSlider* slider = new QSlider(Qt::Horizontal, mainwindow);
slider->setRange(0, 100);
QSpinBox* spinBox = new QSpinBox(mainwindow);
spinBox->setRange(0, 100);
InterClass* interClass = new InterClass(mainwindow, slider, spinBox);
QObject::connect(slider, SIGNAL(valueChanged(int)), interClass, SLOT(setValue(int)));
QObject::connect(spinBox, SIGNAL(valueChanged(int)), interClass, SLOT(setValue(int)));
mainlayout->addWidget(slider);
mainlayout->addWidget(spinBox);
mainwindow->setLayout(mainlayout);
mainwindow->show();
return app.exec();
}