In this example, I will create a Qt4 GUI Application to change color inside a rectangle using three slider.
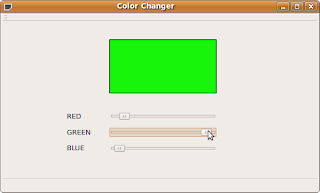
Create a new Qt4 GUI Application in Qt Creator.
Use Qt Designer to edit mainwindow.ui, add three Horizontal Slider, named redSlider, greenSlider and blueSlider and place them as the picture.
Set QAbstractSlider>maximum to 255.

Modify mainwindow.h and mainwindow.cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QtGui/QMainWindow>
namespace Ui
{
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = 0);
~MainWindow();
private:
Ui::MainWindow *ui;
void paintEvent(QPaintEvent*);
int r, g, b;
private slots:
void rChanged(int);
void gChanged(int);
void bChanged(int);
};
#endif // MAINWINDOW_H
mainwindow.h
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QPainter>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent), ui(new Ui::MainWindow)
{
r = g = b = 0;
ui->setupUi(this);
connect(ui->redSlider, SIGNAL(valueChanged(int)),
this, SLOT(rChanged(int)));
connect(ui->greenSlider, SIGNAL(valueChanged(int)),
this, SLOT(gChanged(int)));
connect(ui->blueSlider, SIGNAL(valueChanged(int)),
this, SLOT(bChanged(int)));
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::rChanged(int tr)
{
r = tr;
update();
}
void MainWindow::gChanged(int tg)
{
g = tg;
update();
}
void MainWindow::bChanged(int tb)
{
b = tb;
update();
}
void MainWindow::paintEvent(QPaintEvent *event)
{
QRect rect(200, 50, 200, 100);
QPainter painter(this);
painter.setRenderHint(QPainter::Antialiasing, true);
painter.setPen(QPen(Qt::black, 1, Qt::SolidLine, Qt::RoundCap));
painter.setBrush(QBrush(QColor::QColor(r, g, b, 255),
Qt::SolidPattern));
painter.drawRect(rect);
}
mainwindow.cpp
Save ALL, Re-build and Run, it should be work.