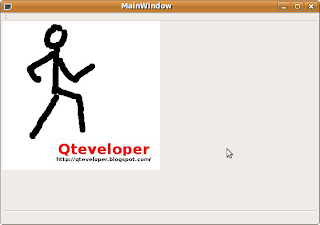
Create a Qt4 GUI Application, download the picture and save in the project directory, named "qtman.jpg".
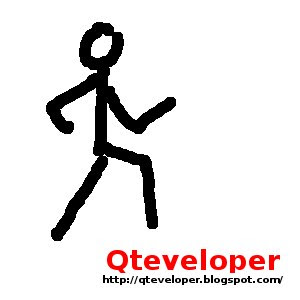
Modify mainwindow.h and mainwindow.cpp
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QtGui/QMainWindow>
namespace Ui
{
class MainWindow;
}
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = 0);
~MainWindow();
private:
Ui::MainWindow *ui;
void paintEvent(QPaintEvent*);
};
#endif // MAINWINDOW_H
mainwindow.h
#include "mainwindow.h"
#include "ui_mainwindow.h"
#include <QPainter>
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent), ui(new Ui::MainWindow)
{
ui->setupUi(this);
}
MainWindow::~MainWindow()
{
delete ui;
}
void MainWindow::paintEvent(QPaintEvent *event)
{
QImage image("qtman.jpg");
QRect rect(0, 0, image.width(), image.height()); //define the area used to display
QPainter painter(this);
painter.drawImage(rect, image);
}
mainwindow.cpp